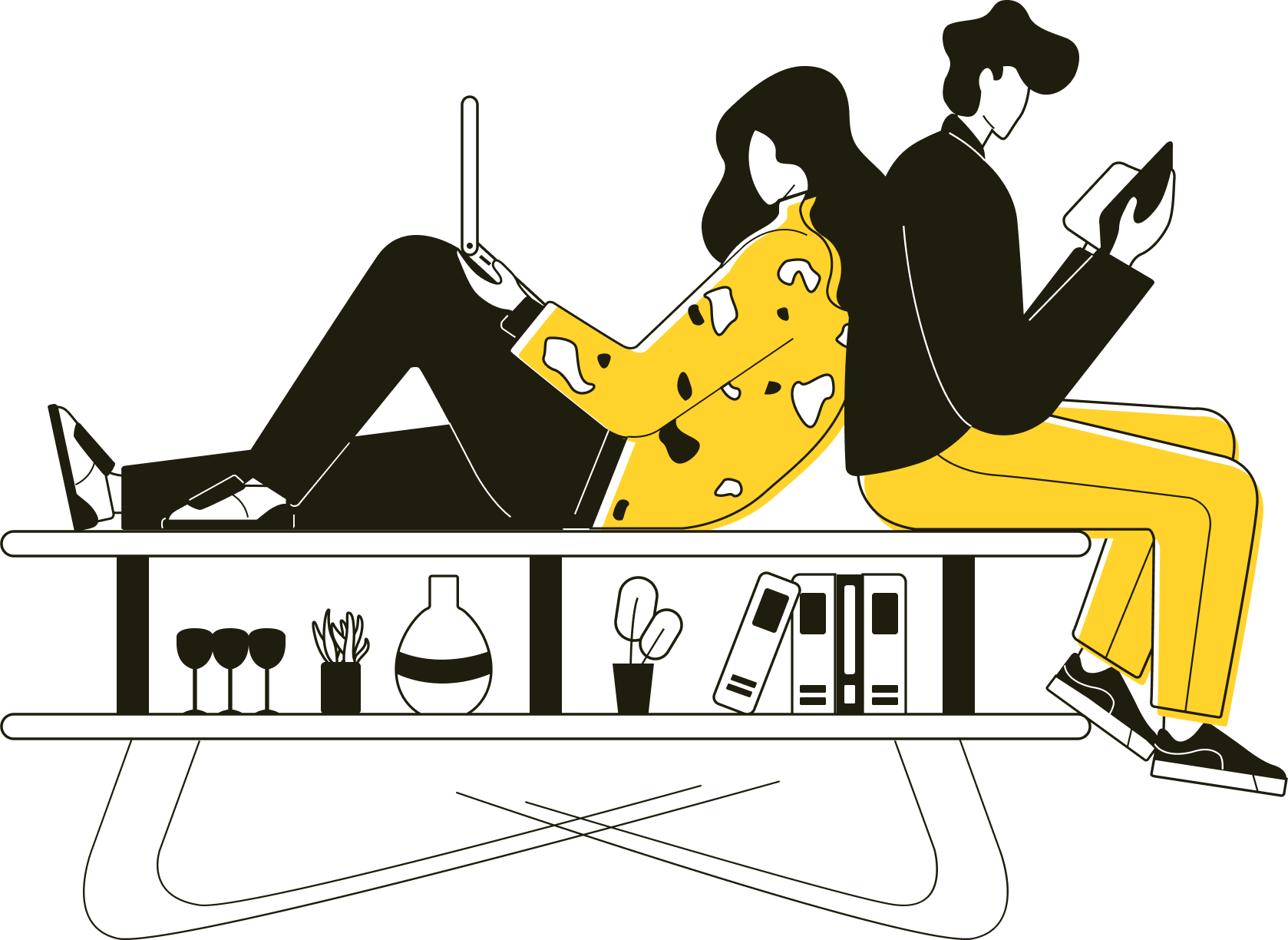
Oops! Page Not Found
Apologies, the page you are seeking may no longer be available, its name might have been altered, or it could be temporarily inaccessible. Kindly verify the URL or simply go back to the homepage
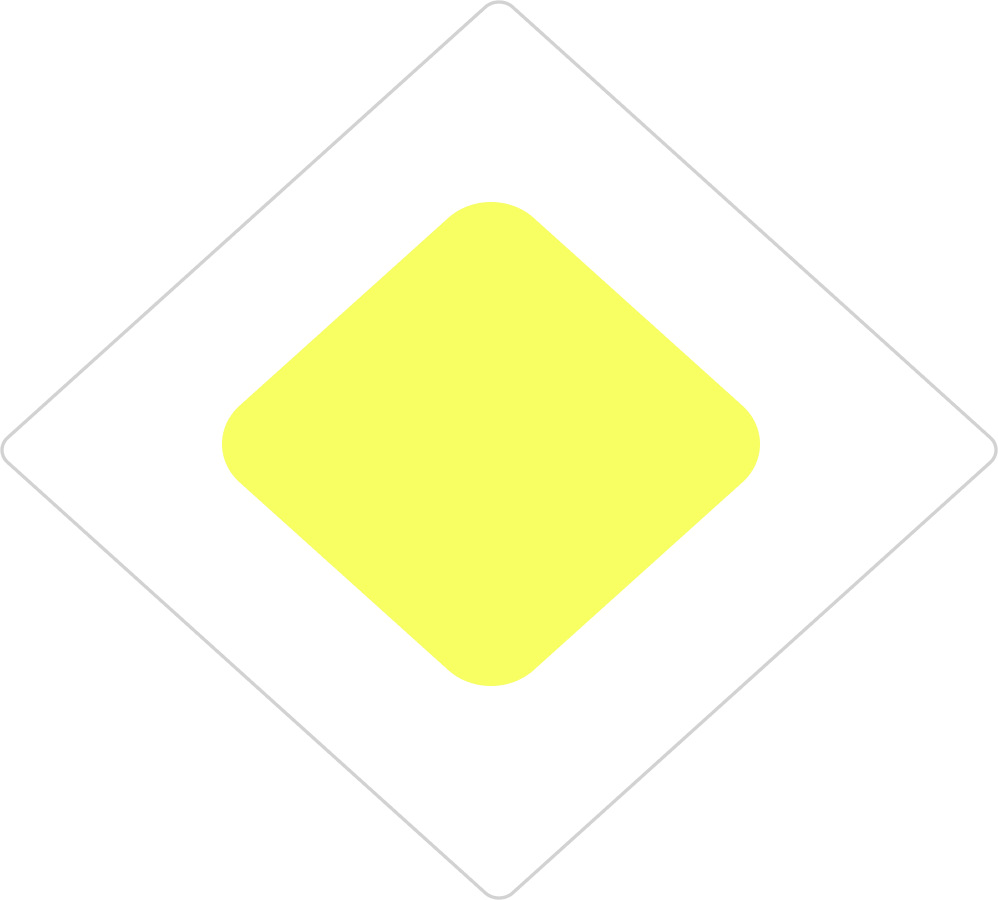
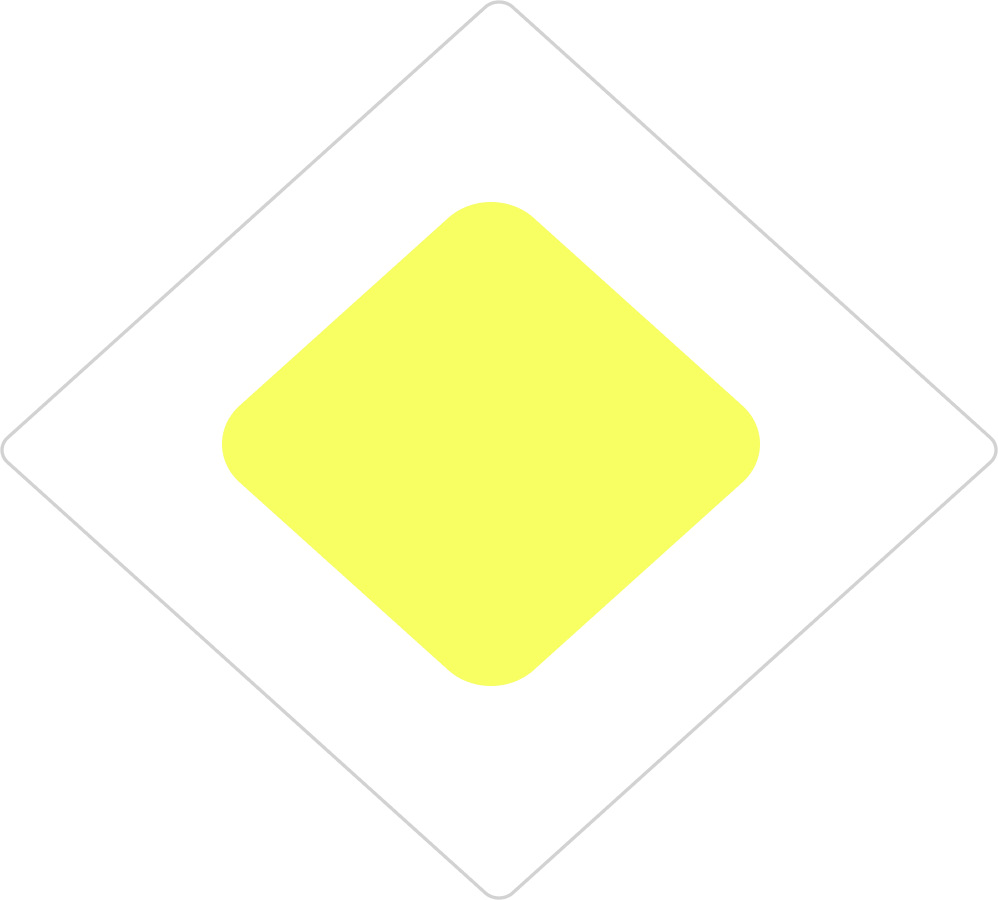
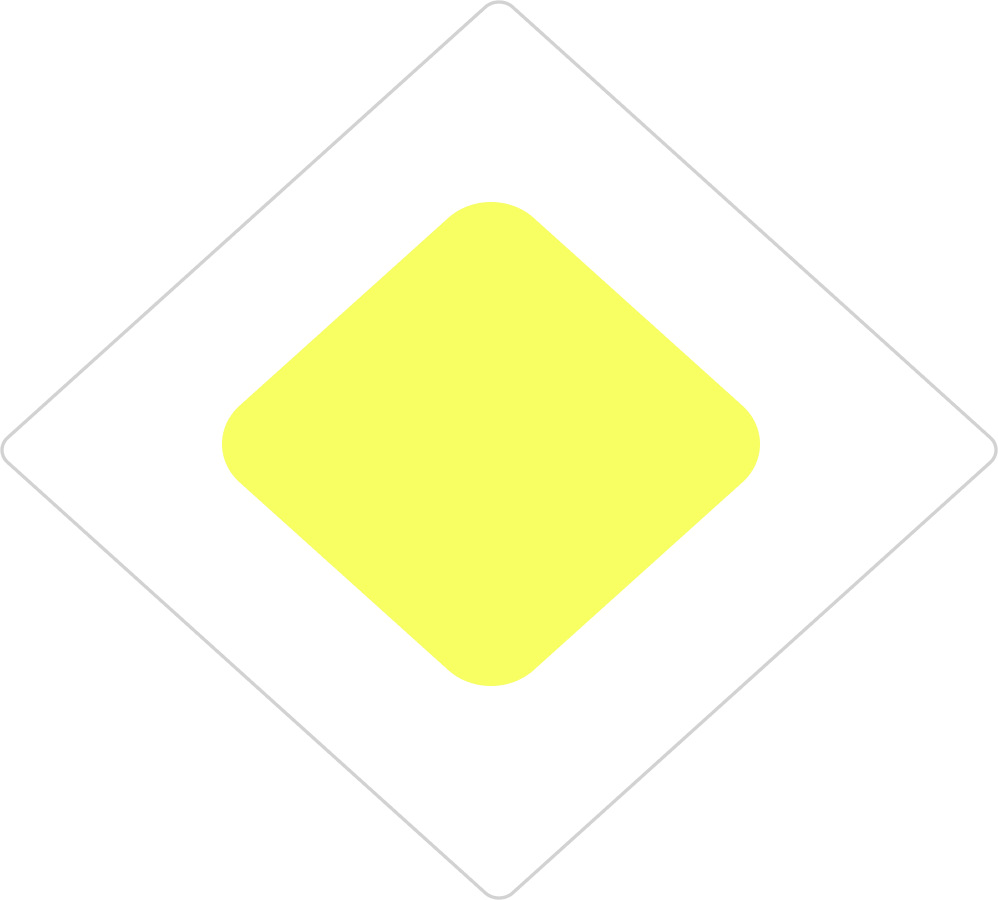
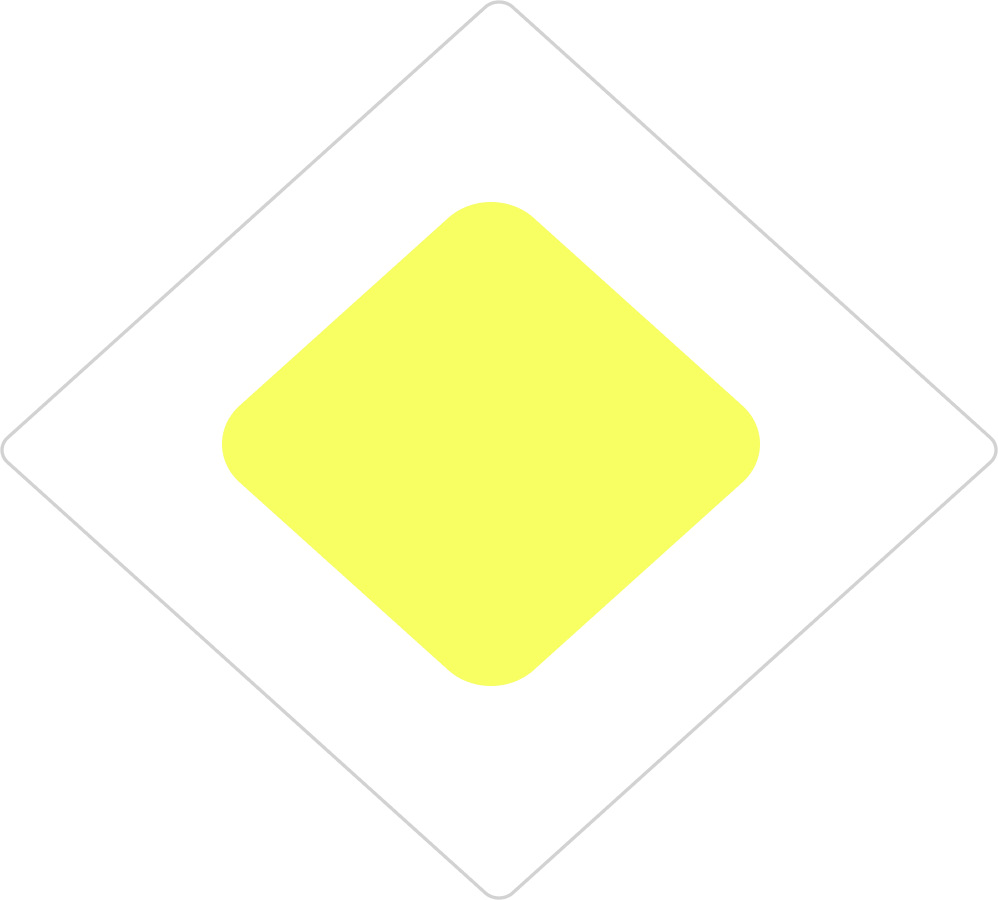
Apologies, the page you are seeking may no longer be available, its name might have been altered, or it could be temporarily inaccessible. Kindly verify the URL or simply go back to the homepage